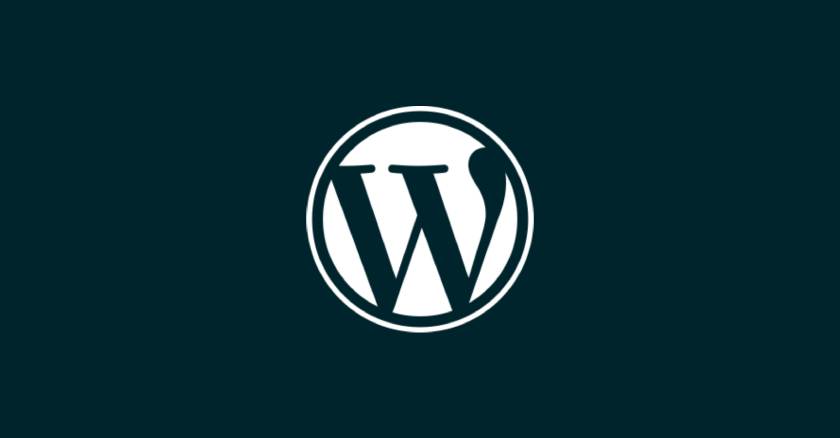
Custom Elementor Widget for Displaying Taxonomies
This guide explains how to create a custom Elementor widget to display post taxonomies with customizable styles and text settings.
Introduction
This code creates a custom Elementor widget under the namespace projectsengine
. It allows users to display taxonomies such as categories or tags for the current post, with options to customize the text before the taxonomy terms and control the appearance of the tags.
Namespace and Widget Setup
- The widget class is defined within the
projectsengine
namespace. - The class extends
Widget_Base
, a core Elementor class. - The widget name, title, icon, category, and keywords are defined using the
get_name()
,get_title()
,get_icon()
,get_categories()
, andget_keywords()
methods.
Controls Setup
The register_controls()
method defines the widget’s controls:
- Content Section: Includes settings for selecting the taxonomy (categories, tags, etc.) and for adding text before the list of terms.
- Style Section: Allows customization of tag styles, including background color, text color, padding, border radius, typography, and spacing between tags.
Getting Available Taxonomies
The get_available_taxonomies()
function retrieves all taxonomies available on the site, returning them as options for the user to select.
Rendering the Widget
The render()
method outputs the selected taxonomy terms of the current post as clickable links. It uses WordPress functions like get_the_terms()
and get_term_link()
to fetch and display terms.
Customization Options
- Text before categories: Custom text that appears before the taxonomy terms.
- Tag styles: Full control over tag design, including typography, colors, and spacing.
This widget offers a highly customizable way to display taxonomies for posts in Elementor with a sleek and adjustable design.
<?php
namespace projectsengine;
use Elementor\Plugin;
use Elementor\Widget_Base;
use Elementor\Controls_Manager;
use Elementor\Group_Control_Typography;
class Taxonomies extends Widget_Base {
public function get_name() {
return 'taxonomies_widget';
}
public function get_title() {
return __('ProjectsEngine - Taxonomies', 'projectsengine');
}
public function get_icon() {
return 'eicon-taxonomy-filter';
}
public function get_categories() {
return ['projectsengine'];
}
public function get_keywords() {
return ['taxonomy', 'categories', 'terms'];
}
protected function register_controls() {
// Content Section
$this->start_controls_section(
'content_section',
[
'label' => __('Settings', 'projectsengine'),
'tab' => Controls_Manager::TAB_CONTENT,
]
);
$this->add_control(
'taxonomy',
[
'label' => __('Taxonomy', 'projectsengine'),
'type' => Controls_Manager::SELECT,
'options' => $this->get_available_taxonomies(),
'default' => 'category',
]
);
$this->add_control(
'text_format',
[
'label' => __('Text Before Categories', 'projectsengine'),
'type' => Controls_Manager::TEXT,
'default' => __('Categories: ', 'projectsengine'),
]
);
$this->end_controls_section();
// Style Section for Tags
$this->start_controls_section(
'style_section',
[
'label' => __('Tag Style', 'projectsengine'),
'tab' => Controls_Manager::TAB_STYLE,
]
);
$this->add_control(
'tag_background_color',
[
'label' => __('Tag Background Color', 'projectsengine'),
'type' => Controls_Manager::COLOR,
'default' => '#7BC043',
'selectors' => [
'{{WRAPPER}} .taxonomy-tag' => 'background-color: {{VALUE}};',
],
]
);
$this->add_control(
'tag_text_color',
[
'label' => __('Tag Text Color', 'projectsengine'),
'type' => Controls_Manager::COLOR,
'default' => '#ffffff',
'selectors' => [
'{{WRAPPER}} .taxonomy-tag' => 'color: {{VALUE}};',
],
]
);
$this->add_control(
'tag_padding',
[
'label' => __('Padding', 'projectsengine'),
'type' => Controls_Manager::DIMENSIONS,
'size_units' => ['px', 'em'],
'default' => [
'top' => 5,
'right' => 10,
'bottom' => 5,
'left' => 10,
'unit' => 'px',
],
'selectors' => [
'{{WRAPPER}} .taxonomy-tag' => 'padding: {{TOP}}{{UNIT}} {{RIGHT}}{{UNIT}} {{BOTTOM}}{{UNIT}} {{LEFT}}{{UNIT}};',
],
]
);
$this->add_control(
'tag_border_radius',
[
'label' => __('Border Radius', 'projectsengine'),
'type' => Controls_Manager::SLIDER,
'size_units' => ['px', '%'],
'range' => [
'px' => [
'min' => 0,
'max' => 50,
],
'%' => [
'min' => 0,
'max' => 100,
],
],
'default' => [
'unit' => 'px',
'size' => 20,
],
'selectors' => [
'{{WRAPPER}} .taxonomy-tag' => 'border-radius: {{SIZE}}{{UNIT}};',
],
]
);
// Typography Settings for Tags
$this->add_group_control(
Group_Control_Typography::get_type(),
[
'name' => 'tag_typography',
'label' => __('Typography', 'projectsengine'),
'selector' => '{{WRAPPER}} .taxonomy-tag',
]
);
// Control for spacing between tags
$this->add_control(
'tag_spacing',
[
'label' => __('Spacing Between Tags', 'projectsengine'),
'type' => Controls_Manager::SLIDER,
'range' => [
'px' => [
'min' => 0,
'max' => 50,
],
],
'selectors' => [
'{{WRAPPER}} .taxonomy-tag' => 'margin-right: {{SIZE}}px;',
],
'default' => [
'unit' => 'px',
'size' => 10,
],
]
);
$this->end_controls_section();
}
// Function to get available taxonomies
protected function get_available_taxonomies() {
$taxonomies = get_taxonomies([], 'objects');
$options = [];
foreach ($taxonomies as $taxonomy) {
$options[$taxonomy->name] = $taxonomy->label;
}
return $options;
}
protected function render() {
$settings = $this->get_settings_for_display();
$taxonomy = $settings['taxonomy'];
$text_format = $settings['text_format'];
// Get the terms for the current post based on the specified taxonomy
$terms = get_the_terms(get_the_ID(), $taxonomy);
if ($terms && !is_wp_error($terms)) {
// Start the div with the formatted text
echo '<div class="taxonomy-text">' . esc_html($text_format);
// Loop through each term and display it as a link
foreach ($terms as $term) {
// Get the term link (URL)
$term_link = get_term_link($term);
// Check if the term link is valid
if (!is_wp_error($term_link)) {
// Output the term as a clickable link
echo '<a href="' . esc_url($term_link) . '" class="taxonomy-tag">' . esc_html($term->name) . '</a>';
}
}
// Close the div
echo '</div>';
}
}
protected function content_template() {}
}
Plugin::instance()->widgets_manager->register( new Taxonomies() );