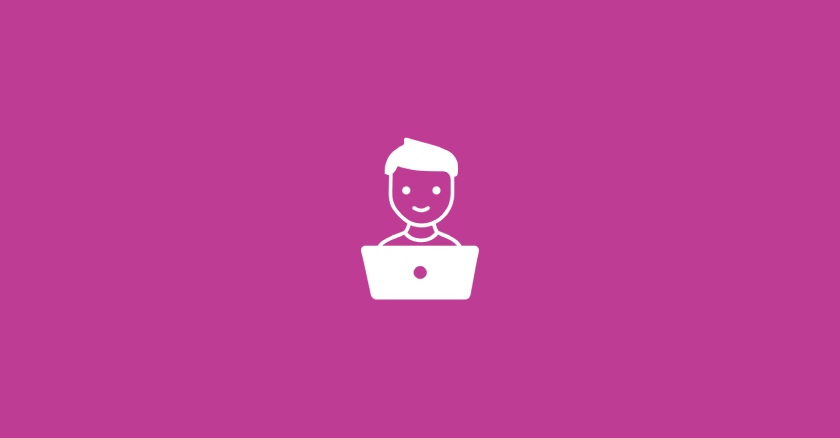
Enhancing WordPress Core Blocks with Custom Attributes
Adding custom attributes is a powerful way to enhance flexibility and control.
In the ever-evolving landscape of WordPress development, customization is key. For developers seeking to extend the functionality of core blocks in the Gutenberg editor, adding custom attributes is a powerful way to enhance flexibility and control. This article outlines how to implement custom attributes such as padding and maximum width to core blocks using JavaScript and PHP, specifically tailored for your WordPress projects.
Adding Custom Attributes to Core Blocks
First, we need to introduce custom attributes to all core blocks. This involves using the WordPress hooks and filters to modify the block settings.
Step 1: Define Custom Attributes
We start by defining our custom attributes using the addFilter
function from the wp.hooks
package. These attributes include paddingTop
, paddingBottom
, and maxWidth
.
const { addFilter } = wp.hooks;
const { createHigherOrderComponent } = wp.compose;
const { InspectorControls } = wp.blockEditor;
const { PanelBody, RangeControl } = wp.components;
// Add custom attributes to all core blocks
const addCustomAttributes = (settings) => {
if (settings.name.startsWith('core/')) {
settings.attributes = {
...settings.attributes,
paddingTop: {
type: 'number',
default: 0,
},
paddingBottom: {
type: 'number',
default: 0,
},
maxWidth: {
type: 'number',
default: 1240,
},
};
}
return settings;
};
addFilter(
'blocks.registerBlockType',
'zing/zing-custom-attributes',
addCustomAttributes
);
Step 2: Create Inspector Controls
Next, we create a higher-order component (HOC) to add controls for these attributes in the block’s Inspector Panel. This allows users to adjust the padding and max width directly from the editor.
// Create a higher-order component to add controls to the Inspector Panel
const withInspectorControls = createHigherOrderComponent((BlockEdit) => {
return (props) => {
if (props.name.startsWith('core/') && props.name !== 'core/columns') {
const {
attributes: { paddingTop, paddingBottom, maxWidth },
setAttributes,
} = props;
return (
<>
<BlockEdit {...props} />
<InspectorControls>
<PanelBody title="Custom Settings" initialOpen={true}>
<RangeControl
label="Padding Top"
value={paddingTop}
onChange={(value) => setAttributes({ paddingTop: value })}
min={0}
max={100}
/>
<RangeControl
label="Padding Bottom"
value={paddingBottom}
onChange={(value) => setAttributes({ paddingBottom: value })}
min={0}
max={100}
/>
<RangeControl
label="Max Width"
value={maxWidth}
onChange={(value) => setAttributes({ maxWidth: value })}
min={0}
max={2000}
/>
</PanelBody>
</InspectorControls>
</>
);
}
return <BlockEdit {...props} />;
};
}, 'withInspectorControls');
addFilter(
'editor.BlockEdit',
'zing/with-inspector-controls',
withInspectorControls
);
Wrapping Block Content with Custom Styles
To apply the custom attributes to the rendered block content, we utilize a PHP function. This function modifies the block’s HTML output by wrapping it with a div
that applies the specified padding and max-width styles.
Step 3: Define the PHP Wrapper Function
The PHP function zing_block_wrapper
filters the block content and adds a wrapper with custom styles based on the block’s attributes.
if ( !function_exists( 'zing_block_wrapper' ) ) {
/**
* Filter blocks.
*
* @param string $block_content
* @param array $block
* @return string
*/
function zing_block_wrapper( $block_content, $block ) {
if ( strpos( $block['blockName'], 'core/' ) === 0 && $block['blockName'] !== 'core/columns' ) {
// Extract the block type (e.g., 'paragraph', 'heading')
$block_type = str_replace( 'core/', '', $block['blockName'] );
// Get custom attributes
$padding_top = isset( $block['attrs']['paddingTop'] ) ? $block['attrs']['paddingTop'] : 0;
$padding_bottom = isset( $block['attrs']['paddingBottom'] ) ? $block['attrs']['paddingBottom'] : 0;
$max_width = isset( $block['attrs']['maxWidth'] ) ? $block['attrs']['maxWidth'] : 1240;
// Build the style attribute
$style = 'style="';
$style .= 'padding-top:' . esc_attr( $padding_top ) . 'px;';
$style .= 'padding-bottom:' . esc_attr( $padding_bottom ) . 'px;';
if ( $max_width > 0 ) {
$style .= 'max-width:' . esc_attr( $max_width ) . 'px;';
}
$style .= 'margin:0 auto;';
$style .= 'padding-left:10px;padding-right:10px;width: 100%;';
$style .= '"';
$content = '<div class="core-block-wrapper">';
$content .= '<div class="core-block-inner core-block-container wp-block-zing-' . esc_attr( $block_type ) . '" ' . $style . '>';
$content .= $block_content;
$content .= '</div>';
$content .= '</div>';
return $content;
}
return $block_content;
}
add_filter( 'render_block', 'zing_block_wrapper', 10, 2 );
}
Conclusion
By following these steps, you can effectively extend the functionality of WordPress core blocks to include custom attributes for padding and maximum width. This not only enhances the visual flexibility of your blocks but also provides a more tailored editing experience for users.
Implementing these changes involves both JavaScript for the Gutenberg editor interface and PHP for rendering the final block content. Together, these modifications can significantly enhance the customization capabilities of your WordPress site.
This comprehensive guide should help you get started with adding custom attributes to WordPress core blocks. Happy coding!