Creating WordPress Gutenberg Blocks
Creating Gutenberg blocks is a great way to extend the functionality of the Gutenberg editor and customize the content editing experience for WordPress users.
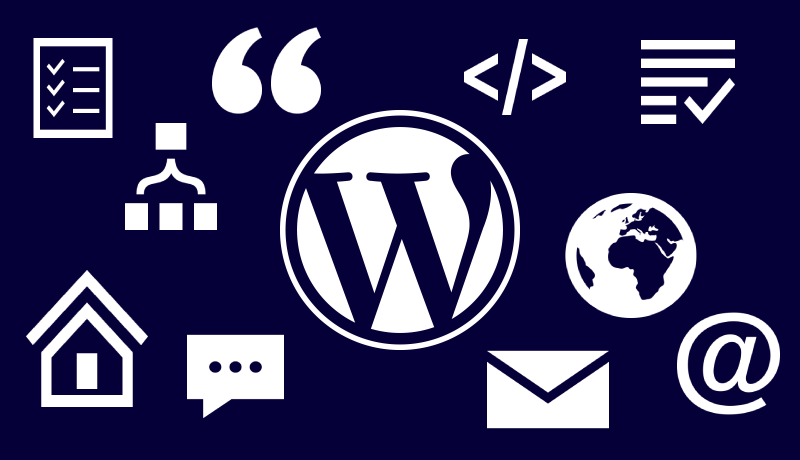
Creating Gutenberg blocks is a great way to extend the functionality of the Gutenberg editor and customize the content editing experience for WordPress users. Here’s how you can create Gutenberg blocks:
Step 1: Set up your development environment
To create Gutenberg blocks, you’ll need a development environment with Node.js and npm installed. You can install Node.js and npm by visiting the official Node.js website and following the installation instructions for your operating system.
Step 2: Create a new WordPress plugin
To create a Gutenberg block, you’ll need to create a new WordPress plugin. You can do this by creating a new folder in the “wp-content/plugins” directory of your WordPress installation and adding a new PHP file with the plugin header information.
Step 3: Install the necessary packages
You’ll need to install the necessary packages to create Gutenberg blocks. These packages include “@wordpress/blocks
“, “@wordpress/components
“, “@wordpress/editor
“, and “@wordpress/i18n
“. You can install these packages by running the following command in your plugin directory:
npm install --save @wordpress/blocks @wordpress/components @wordpress/editor @wordpress/i18n
Step 4: Create a new block
To create a new Gutenberg block, you’ll need to create a new JavaScript file in your plugin directory. In this file, you’ll define the block’s attributes, settings, and content.
Here’s an example of how you can create a simple “Hello World
” block:
import { registerBlockType } from '@wordpress/blocks';
import { TextControl } from '@wordpress/components';
import { __ } from '@wordpress/i18n';
registerBlockType( 'my-plugin/hello-world', {
title: __( 'Hello World', 'my-plugin' ),
icon: 'smiley',
category: 'common',
attributes: {
content: {
type: 'string',
source: 'text',
selector: 'p',
},
},
edit( { attributes, setAttributes } ) {
const { content } = attributes;
function onChangeContent( newContent ) {
setAttributes( { content: newContent } );
}
return (
<div className="my-plugin-hello-world">
<TextControl
label={ __( 'Content', 'my-plugin' ) }
value={ content }
onChange={ onChangeContent }
/>
</div>
);
},
save( { attributes } ) {
const { content } = attributes;
return (
<p>{ content }</p>
);
},
} );
This block adds a new “Hello World” block to the “Common” category of the Gutenberg editor. When the block is edited, it displays a text input field where the user can enter the block’s content. When the block is saved, it renders the content as a paragraph.
Step 5: Register the block
Once you’ve defined your block, you’ll need to register it with the Gutenberg editor. You can do this by calling the “registerBlockType()” function and passing in the block’s name and settings.
Step 6: Test your block
To test your block, activate your plugin in WordPress and create a new post or page. You should see your new block in the Gutenberg editor’s block selector. Add the block to your post or page and test it to make sure it works as expected.
Creating Gutenberg blocks can be challenging at first, but with some practice and experimentation, you’ll be able to create custom blocks that enhance the content editing experience for WordPress users.
Was this post helpful? ( Answers: 2 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!