How to Change the Author Base in WordPress Dynamically
The "Author Base" in WordPress is a component of the URL structure that determines how author archives are accessed on a website.
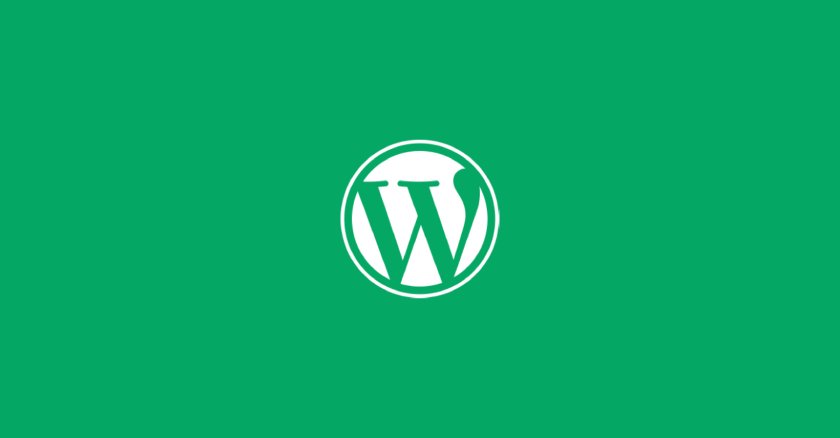
The “Author Base” in WordPress is a component of the URL structure that determines how author archives are accessed on a website. By default, the Author Base is set to “/author/”. For example, if your website’s domain is “example.com”, and you have an author named “John”, their author archive URL would be “example.com/author/john/”.
Unlike the category or tag bases in WordPress, which can be easily customized through the Permalinks Settings screen in the WordPress admin area, the Author Base does not have an option for direct modification. This means that, by default, it cannot be changed through the Permalinks settings page.
However, if you wish to customize the Author Base for your WordPress site, you can achieve this through alternative methods such as using plugins or custom code. There are various plugins available that provide functionality to modify the Author Base according to your preferences. Alternatively, you can implement custom code solutions tailored to your specific needs.
In the context of WordPress development, changing the Author Base programmatically involves modifying the URL structure used for author archives. This article presents two approaches to achieve this task.
- Defining the New Base in Source Code: The first approach involves defining the new Author Base directly within the source code of your WordPress theme or plugin. This method offers a straightforward way to set the Author Base statically.
- Dynamic Modification via Permalinks Page: The second approach provides more flexibility by allowing users to modify the Author Base dynamically through an input field on the Permalinks settings page in the WordPress admin area. This method enables users to customize the Author Base without needing to edit the source code directly.
When rewriting the URL structure to change the Author Base, the key is to modify the $wp_rewrite
global variable. This variable represents a single instance of the WP_Rewrite
class in WordPress, responsible for managing URL rewrite rules. By updating the rewrite rules through this variable, WordPress ensures that the changes are applied consistently across the site. This is particularly important for maintaining SEO-friendly Pretty Permalinks, which contribute to better search engine visibility and user experience.
Defining the New Base in Hard Code
if( !function_exists('zing_custom_author_base') ) {
/**
* Custom author base
* Different url: /user/user-nicename instead of /author/user-nicename
*/
function zing_custom_author_base() {
global $wp_rewrite;
$wp_rewrite->author_base = 'user';
}
add_action( 'init', 'zing_custom_author_base' );
}
This code changes the default Author Base in WordPress. Instead of /author/username
, it sets the URL format to /user/username
. It’s hooked to WordPress initialization to ensure it works properly when the site starts.
To make this change permanent, go to the Permalinks admin screen and click Save. Or, you can use flush_rewrite_rules()
to rebuild the permalink structure programmatically, but remember it’s resource-intensive and should only be called once.
Dynamic Modification via Permalinks Page
/**
* Rewrite author base to custom
*
* @return void
*/
function zing_author_base_rewrite() {
global $wp_rewrite;
$author_base = get_option( 'zing_author_base' );
if ( !empty( $author_base ) ) {
$wp_rewrite->author_base = $author_base;
}
else {
$wp_rewrite->author_base = 'user';
}
}
add_action( 'init', 'zing_author_base_rewrite' );
/**
* Render textinput for Author base
* Callback for the add_settings_function()
*
* @return void
*/
function zing_author_base_render_field() {
global $wp_rewrite;
printf( '<input name="zing_author_base" id="zing_author_base" type="text" value="%s" class="regular-text code">', esc_attr( $wp_rewrite->author_base ));
}
/**
* Add a setting field for Author Base to the "Optional" Section
* of the Permalinks Page
*
* @return void
*/
function zing_author_base_add_settings_field() {
add_settings_field(
'zing_author_base',
esc_html__( 'Author base', ZING_TEXT_DOMAIN ),
'zing_author_base_render_field',
'permalink',
'optional',
array( 'label_for' => 'zing_author_base' )
);
}
add_action( 'admin_init', 'zing_author_base_add_settings_field' );
/**
* Sanitize and save the given Author Base value to the database
*
* @return void
*/
function zing_author_base_update() {
$author_base = get_option( 'zing_author_base' );
if ( isset( $_POST['zing_author_base'] ) && isset( $_POST['permalink_structure'] ) && check_admin_referer( 'update-permalink' ) ) {
$base = sanitize_title( $_POST['zing_author_base'] );
if ( empty( $base ) ) {
add_settings_error(
'zing_author_base',
'zing_author_base',
esc_html__( 'Invalid author base.', ZING_TEXT_DOMAIN ),
'error'
);
} elseif ( $author_base != $base ) {
update_option( 'zing_author_base', $base );
}
}
}
add_action( 'admin_init', 'zing_author_base_update' );
This code snippet accomplishes a few tasks related to customizing the Author Base in WordPress:
- The
zing_author_base_rewrite()
function modifies the Author Base according to the value set in the “zing_author_base” option. If the option is empty, it defaults to ‘user’. - The
zing_author_base_render_field()
function renders a text input field for the Author Base setting on the Permalinks settings page in the WordPress admin area. - The
zing_author_base_add_settings_field()
function adds a setting field for the Author Base to the “Optional” section of the Permalinks settings page. - The
zing_author_base_update()
function sanitizes and saves the provided Author Base value to the database when the Permalinks settings are updated in the admin area.
Together, these functions enable users to customize the Author Base through the WordPress admin interface and ensure that the changes are reflected in the site’s permalink structure.
Here’s an explanation of each function used in the code snippet:
- add_settings_error: This function is used to add an error or an update message to the settings page. It takes parameters such as the setting ID, an error code, the error message, and the error type (e.g., ‘error’, ‘updated’). In the context of the code snippet, it’s used to display an error message if the provided author base is invalid.
- update_option: This function updates the value of a specific option in the WordPress options table. It takes two parameters: the option name and the new option value. In the code snippet, it’s used to update the ‘zing_author_base’ option with the new author base value.
- add_settings_field: This function adds a new field to a settings page in the WordPress admin area. It’s typically used in conjunction with the
register_setting
function to add custom settings fields. It takes parameters such as the field ID, the field label, a callback function to render the field, the settings page slug, the settings section ID, and additional arguments. In the code snippet, it’s used to add a field for the custom author base to the Permalinks settings page. - get_option: This function retrieves the value of a specific option from the WordPress options table. It takes the option name as a parameter and returns the option value. In the code snippet, it’s used to retrieve the current value of the ‘zing_author_base’ option.
These functions are essential for managing settings and options in WordPress and are commonly used in plugin and theme development to provide customizable features to users.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!