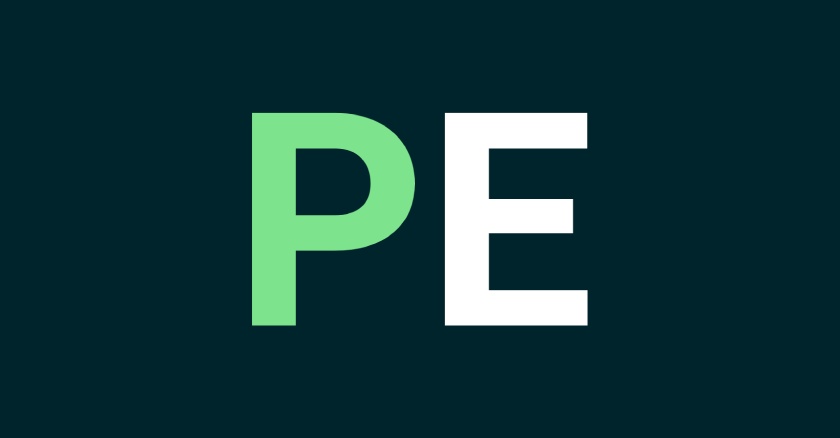
jsx
So, JSX, ever heard of it? It's like this special sauce for JavaScript that lets you toss in HTML-like tags right into your code.
So, JSX, ever heard of it? It’s like this special sauce for JavaScript that lets you toss in HTML-like tags right into your code. It’s a big hit with React fans, ’cause it makes writing UI stuff a breeze.
Here’s the deal:
- React likes to mix things up, you know? It throws the logic and the markup together in these things called components. So instead of having your HTML and JavaScript in separate corners of the room, they’re all cozy in one spot.
- Each of these components is just a fancy JavaScript function that spits out some HTML-ish stuff for React to show on the screen. And JSX? Well, it’s what makes that HTML-ish stuff look, well, like HTML.
Now, about bringing your good ol’ HTML into the JSX club:
You’ve got this solid chunk of HTML, right?
<div class="sidebar">
<h2>Sidebar</h2>
<ul>
<li>Home</li>
<li>About</li>
<li>Contact</li>
</ul>
</div>
And you’re itching to sprinkle it into your JSX component:
export default function Sidebar() {
return (
<div className="sidebar">
<h2>Sidebar</h2>
<ul>
<li>Home</li>
<li>About</li>
<li>Contact</li>
</ul>
</div>
);
}
JSX has some quirks you gotta roll with:
- Wrap it up: JSX wants all your tags snuggled up inside one parent tag or a Fragment.
- Close the door: Don’t forget to close all your tags, even the self-closing ones.
- camelCase it: Those attributes? Yeah, make ’em camelCase. JavaScript’s picky about that stuff.
Stick to these simple rules, maybe use a JSX converter if you’re feeling lazy, and you’ll be whipping up JSX like a pro in no time.
So, JSX: making JavaScript taste a little more like HTML, one tag at a time. Easy peasy!