WordPress Hooks: How to Create Custom WordPress Hooks
If you're a WordPress developer, it's a good idea to give other developers a way to interact with your code. Custom hooks enable you to do just that. They provide other developers the ability to enhance and alter the fu...
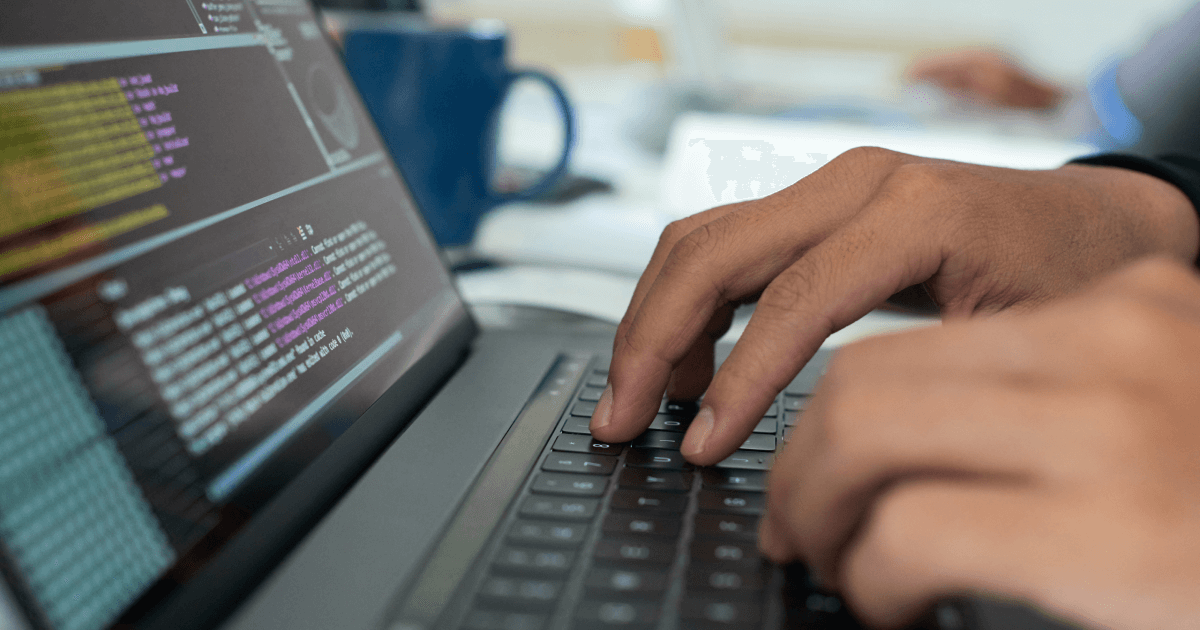
If you’ve read our article about how to use actions and filters you probably know how you can use the hooks to integrate your custom code into WordPress runtime.
If you’re a WordPress developer, it’s a good idea to give other developers a way to interact with your code. Custom hooks enable you to do just that. They provide other developers the ability to enhance and alter the functionality of your plugins and themes.
Creating your own actions and filters is a straightforward process. You utilize the same hook-creation methods that WordPress Core does.
Custom Action Hooks in WordPress
This is very simple. You just use the do_action() function to create custom action hooks in WordPress.
<?php
do_action( 'custom_action' );
Other developers may now connect to your plugin or theme without having to edit the source code.
Make sure to properly document your custom hooks, outlining what they perform in detail. After all, the primary purpose of writing custom hooks is to allow other developers to modify your code.
Custom Filter Hooks in WordPress
This is very simple. You just use the apply_filters() function to create custom filter hooks in WordPress.
The parameters of your custom filter should include a unique name and a value to filter. Other developers can use the add_filter() function to hook into your custom filter and alter the given value.
<?php
$value = "This is a random string, but it can be any data type";
// filters modify a value
apply_filters( 'custom_filter', $value );
Naming Convention
It’s critical to give each of your custom hooks a distinct name. Because each plugin or theme might have its own set of custom hooks, having similar hook names can result in code conflicts with unexpected effects.
For example, if you call your action send_email_to_user, other developers are likely to use the same phrase because it isn’t distinctive enough. If a website downloads both your and other developers’ plugins, it may result in issues that are difficult to track down.
WooCommerce Custom Hooks
Individual WordPress plugins and themes can benefit from a large ecosystem of expandable plugins thanks to custom hooks. Take, for example, the WooCommerce plugin. It not only provides e-commerce capabilities to WordPress, but it also contains a plethora of hooks within its code.
WooCommerce offers hundreds of extensions that use hooks to extend and improve its basic functionality.
You may use these extensions to connect WooCommerce to services like Stripe, MailChimp, Salesforce, and Zapier, among others.
Custom Hooks: When to use them?
Depending on the project you’re designing and who it’s meant for, you may question if any custom hooks are required.
When determining whether or not to add custom hooks, a decent rule of thumb is to see if they provide any extensibility advantages to other developers. If not, it’s best to wait until other developers urge you to include them.
Learn more
WordPress Hooks: How to use actions and filters
Summary
Hooks allow you to not only edit or expand the basic functionality of WordPress but also to modify plugins or themes and allow other developers to change them.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!