React: Building powerful user interfaces with confidence
React is a popular JavaScript library for building user interfaces. It was developed and maintained by Facebook. React allows developers to create interactive and dynamic user interfaces efficiently.
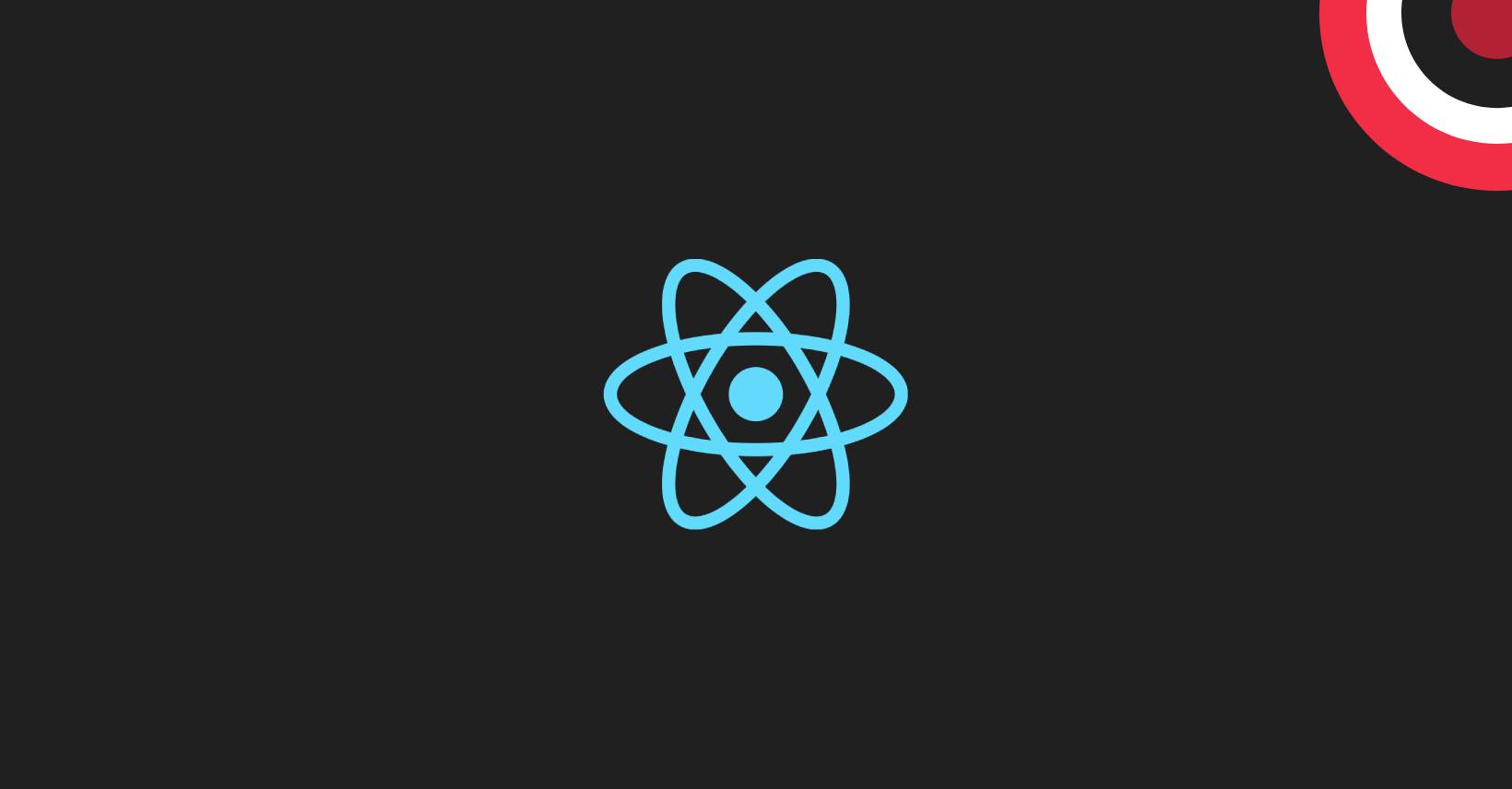
React is a popular JavaScript library for building user interfaces. It was developed and maintained by Facebook. React allows developers to create interactive and dynamic user interfaces efficiently.
Components in React
React follows a component-based architecture. UIs are built using independent and reusable components. Each component encapsulates its logic and can be composed to create complex interfaces.
Components in React are the building blocks of the user interface. You can create a component by defining a JavaScript function or class that returns JSX (React’s syntax extension for JavaScript).
// Functional Component
function MyComponent() {
return <p>This is my component</p>;
}
// Class Component
class MyClassComponent extends React.Component {
render() {
return <p>This is a class component</p>;
}
}
In React, component names must start with a capital letter to distinguish them from regular HTML elements. This naming convention is crucial for React to differentiate between custom components and standard HTML elements. It helps React render and manage components in the virtual DOM properly. Keep in mind this convention when creating and using your React components.
React allows you to compose UIs by nesting components within each other. You can use custom components inside other components, just like you use HTML elements.
function App() {
return (
<div>
<Header />
<MainContent />
<Footer />
</div>
);
}
Writing markup with JSX
Writing markup with JSX is a fundamental aspect of React development. Here’s a brief guide:
- Understanding JSX:
- JSX (JavaScript XML) is a syntax extension for JavaScript commonly used with React.
- It looks similar to XML/HTML but is used within JavaScript code.
- Creating Elements:
- You can use JSX to create React elements. For example:
const element = <h1>Hello, JSX!</h1>;
- Embedding Expressions:
- JSX allows embedding JavaScript expressions inside curly braces
{}
. const name = "John"; const element = <p>Hello, {name}!</p>;
- JSX allows embedding JavaScript expressions inside curly braces
- Rendering JSX Elements:
- To render JSX elements, you can use them in the
ReactDOM.render()
method. const element = <h1>Hello, JSX!</h1>; ReactDOM.render(element, document.getElementById("root"));
- To render JSX elements, you can use them in the
- JSX and HTML Attributes:
- JSX uses camelCase for HTML attributes, like
className
instead ofclass
. const element = <div className="container">Content</div>;
- JSX uses camelCase for HTML attributes, like
- JSX as JavaScript Expressions:
- JSX is closer to JavaScript than HTML. It gets compiled into JavaScript and is treated as an expression.
const isLoggedIn = true; const greeting = isLoggedIn ? <p>Welcome back!</p> : <p>Please log in.</p>;
- Using JSX in Components:
- JSX is commonly used within React components to describe the component’s UI.
function MyComponent() { return <h2>This is a JSX component!</h2>; }
Remember to include the necessary imports, such as React
and ReactDOM
, and ensure that your JSX code gets transpiled into JavaScript using a tool like Babel.
How to display data
Use state or props to display dynamic data in your components. State represents the internal data of a component, while props are used to pass data from parent to child components.
How to render conditions and lists
Conditional rendering allows you to show or hide components based on certain conditions. Mapping over arrays to dynamically render lists of components is a common practice in React.
How to respond to events and update the screen
Use event handlers to respond to user interactions like clicks or input changes. Update the component state to trigger re-renders and reflect changes on the screen.
How to share data between components
If multiple components need access to the same data, move the state to a common ancestor. Use props to pass data from parent components to child components. State management libraries like Redux or context API can be explored for more complex scenarios.
// ParentComponent.js
import React, { useState } from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
const [data, setData] = useState("Hello from Parent!");
const handleButtonClick = () => {
setData("Updated data!");
};
return (
<div>
<ChildComponent message={data} />
<button onClick={handleButtonClick}>Update Data</button>
</div>
);
}
// ChildComponent.js
import React from 'react';
function ChildComponent(props) {
return <p>{props.message}</p>;
}
export default ParentComponent;
This example covers creating, nesting, displaying data, responding to events, and sharing data between components.
*transpiled: Transpilation is the process of converting source code from one programming language to another at the same abstraction level. It’s a term often used in the context of JavaScript development.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!