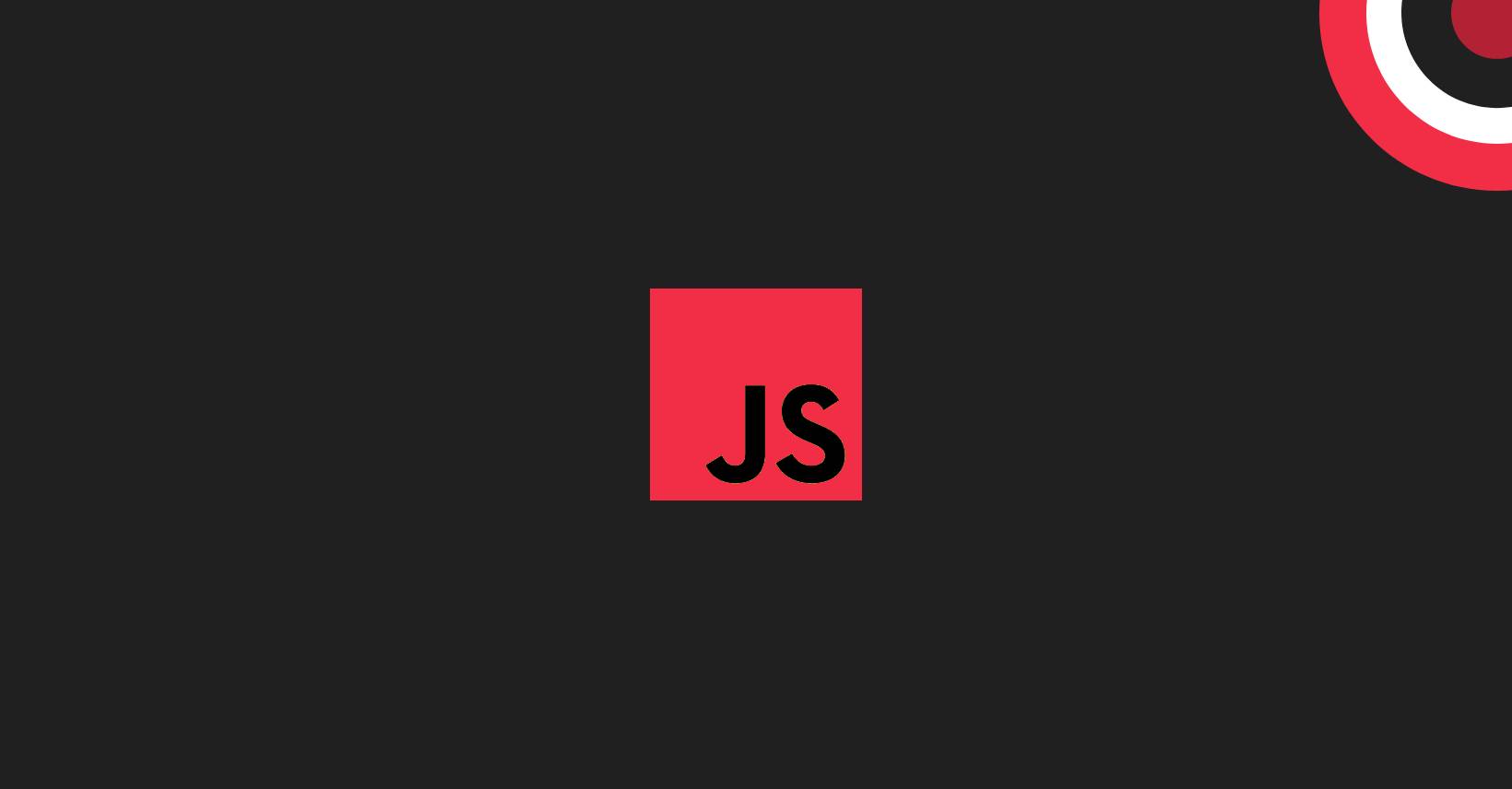
JavaScript modules: Magic for Organized and Efficient Code
As your JavaScript projects grow, those sprawling .js files can become unmanageable monsters. Fear not, aspiring coders, for JavaScript modules arrive to slay the code complexity dragon.
As your JavaScript projects grow, those sprawling .js
files can become unmanageable monsters. Fear not, aspiring coders, for JavaScript modules arrive to slay the code complexity dragon! Let’s explore how these magical tools break down your code into bite-sized chunks, keeping your projects organized and efficient.
The Problem: Code Monsters on the Loose
Imagine a script file growing out of control, like a hungry monster devouring lines of code. Functions start to intertwine, dependencies become cryptic, and debugging becomes a nightmarish quest. This is the reality of large, monolithic scripts – a breeding ground for errors and frustration.
The Solution: Enter the JavaScript Module Knights!
Just like brave knights dividing and conquering a fearsome beast, modules allow you to split your script into separate files, each focused on a specific task. Think of them as neatly organized drawers for your code magic!
Example Time: Backpack Buddies
Consider a script managing a virtual backpack. You can create a backpack.js
file containing the backpack
object and its helper functions. Then, in your main script (say, script.js
), you simply import the backpack
object using the import
keyword. Suddenly, the backpack functionality is available and usable, as if it resided directly in your main script!
// backpack.js
const backpack = {
items: [],
addItem(item) {
this.items.push(item);
console.log(`Added ${item} to the backpack!`);
},
listItems() {
console.log("Backpack contents:");
this.items.forEach(item => console.log(item));
}
};
export default backpack;
// script.js
import backpack from './backpack.js';
// Use the backpack object
backpack.addItem("Notebook");
backpack.addItem("Pen");
backpack.listItems();
<html>
<head>
<script type="module" src="script.js"></script>
<script type="module" src="backpack.js"></script>
</head>
<body>
</body>
</html>
Key Benefits of Modular Magic:
- Since these are modules, they will be automatically deferred by the browser, ensuring optimal page loading and a smooth user experience.
But Wait, There’s More!
Remember, modules are not just for objects. You can package classes, functions, and even constants! Think of them as versatile tools for structuring your code any way you see fit.
A Word of Caution: Scoping Matters
Unlike global variables, modules create their private worlds. Objects defined within a module are not directly accessible from the browser console or other scripts. This keeps your code organized and prevents unexpected conflicts.
The Future of JavaScript: Modules Everywhere!
Mastering modules opens doors to the world of modern JavaScript frameworks like React and Vue.js. These frameworks rely heavily on modules for structuring and organizing code, so understanding them is a valuable skill for any aspiring web developer.
Remember: Embrace the power of JavaScript modules! By breaking down your code into organized, reusable pieces, you’ll conquer code complexity, write cleaner code, and enjoy a smoother development experience.