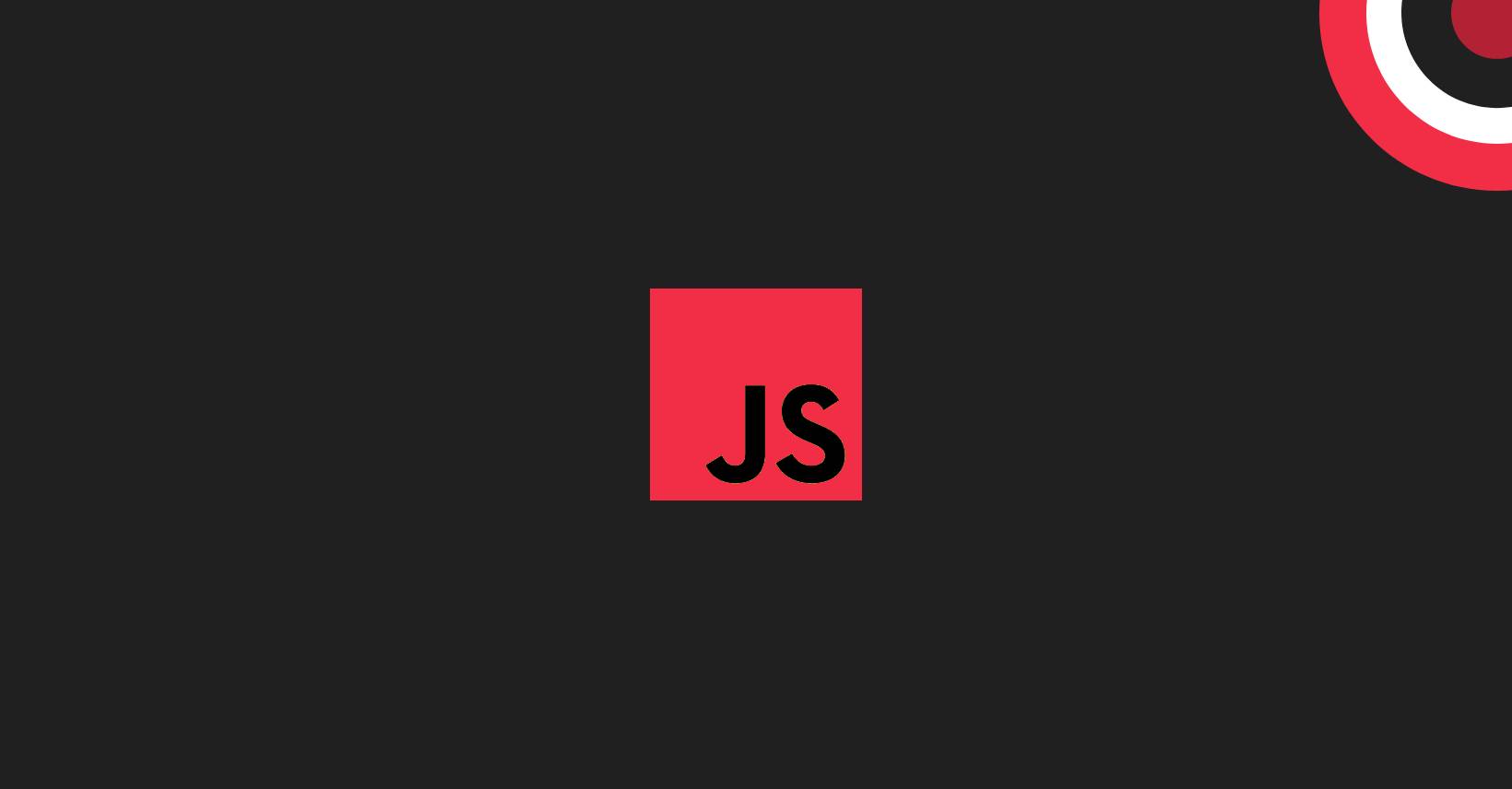
Navigating the JS landscape
Stepping into the world of JavaScript can feel like entering a dense jungle – tangled vines of libraries, frameworks, and acronyms like ECMAScript, TypeScript, and React loom overhead.
Table of Contents
Stepping into the world of JavaScript can feel like entering a dense jungle – tangled vines of libraries, frameworks, and acronyms like ECMAScript, TypeScript, and React loom overhead. Fear not, intrepid explorer! This guide will equip you with a machete and compass to navigate the JavaScript landscape with confidence.
At the Heart of the Jungle: Vanilla JavaScript
Imagine JavaScript as a sturdy tree trunk, supporting the entire ecosystem. This core language, often called “Vanilla JavaScript,” forms the foundation for everything you’ll encounter. It’s the language you write when you write JavaScript, and mastering it is crucial for unlocking the potential of its branches.
ECMAScript: The Official Map
Think of ECMAScript (ES) as the official map of the JavaScript jungle. It’s not the jungle itself, but a detailed description of how browsers should interpret the language. Versions like ES6, ES2015, and beyond represent cutting-edge features, like arrow functions and classes, that push the boundaries of what’s possible.
Dialects of the Jungle: TypeScript and CoffeeScript
Some developers, like seasoned explorers, prefer customized tools. Enter TypeScript and CoffeeScript – dialects of JavaScript that add features or simplify syntax. TypeScript, for example, integrates type annotations for better code organization and error prevention. Look for the telltale “.ts” file extension to spot TypeScript in the wild.
Framework Fortresses: React, Vue, and Angular
Imagine these popular frameworks as fortified settlements within the jungle, offering pre-built structures and tools to accelerate development. React, Vue, and Angular each provide an abstraction layer on top of JavaScript, streamlining tasks like rendering web content and managing data flow. React, for instance, utilizes JSX (JavaScript XML) to seamlessly blend JavaScript and HTML.
Build Tools: Your trusty Machete
Just like a machete clears your path, build tools like Babel, Webpack, and Grunt help transform your human-readable JavaScript into browser-optimized code. These automated helpers handle tasks like minifying code, managing dependencies, and transpiling modern features to older browsers.
Node.js: JavaScript on the Server-Side
In recent years, JavaScript has ventured beyond the browser, colonizing the server with Node.js. This runtime environment allows you to write JavaScript code that runs on the backend of your applications, powering databases, APIs, and more. Get ready to interact with Node.js through tools like NPM (Node Package Manager) when working with modern JavaScript projects.
Your Takeaway: Navigate, Specialize, Conquer
Remember, Vanilla JavaScript is the sturdy trunk supporting all the diverse branches of the JavaScript ecosystem. Mastering this core language is your first step to conquering the jungle. Once you’ve gained your bearings, you can specialize in frameworks, tools, or dialects that align with your interests and goals. With this guide as your compass, you’ll be well on your way to navigating the JavaScript jungle and building web wonders of your own.
Examples:
- Vanilla JavaScript Example: A simple function that logs the current date and time to the console.
function logTime() {
const date = new Date();
console.log(`The current date and time is: ${date}`);
}
logTime();
- ES6 Example: Using arrow functions and arrow functions for conciseness.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(number => number % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
- React Example: A basic React component that displays a welcome message.
function Welcome() {
return <h1>Welcome to the JavaScript Jungle!</h1>;
}
ReactDOM.render(<Welcome />, document.getElementById('root'));
These are just a few examples to illustrate the different aspects of the JavaScript landscape. By exploring and experimenting, you’ll gradually build your map of this vast and exciting ecosystem.