JavaScript Jitters Solved: Debugging Your Code Like a Zen Master
Conquering JavaScript errors? Ditch the panic and channel your inner coding guru with these debugging tips for a calmer, clearer dev journey.
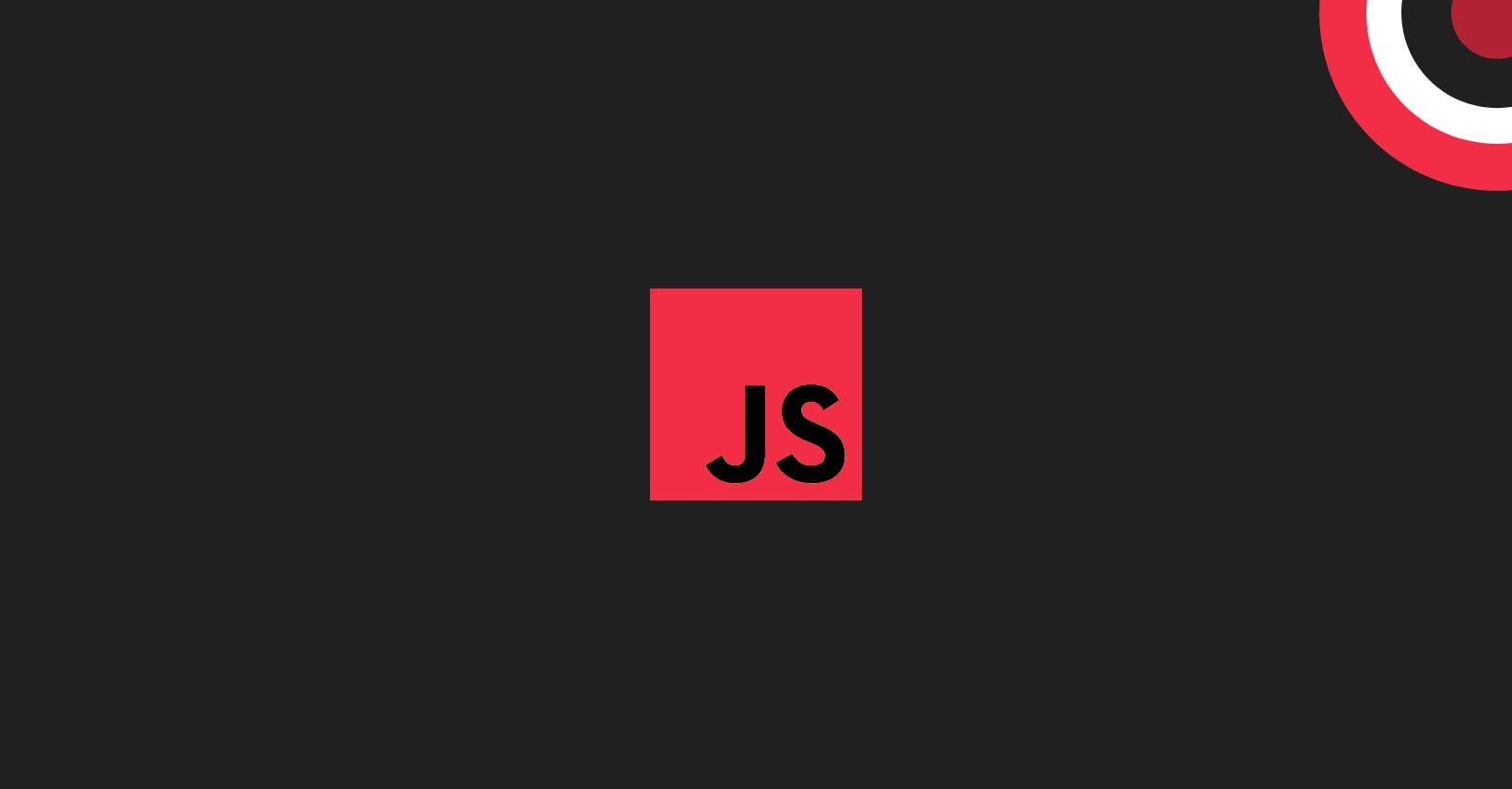
JavaScript: the language that powers dynamic websites, interactive elements, and mind-blowing animations. But for every dazzling result, there’s a shadow lurking – the occasional cryptic error that sends shivers down your spine and coffee cascading into your keyboard. Fear not, fellow coders! Debugging JavaScript doesn’t have to be a frantic caffeine-fueled frenzy. Embrace your inner zen master and unlock the secrets of calm, efficient debugging with these powerful tips:
1. The Error Whisperer
Panicking won’t decipher those cryptic messages. Instead, approach every error with curiosity. It’s not a personal attack, but a puzzle waiting to be solved. Analyze the error message carefully, understanding its type and context. Remember, even the most confusing error holds the key to its resolution.
2. Your Console Concierge
The browser console is your debugging soulmate. Befriend it! Log messages strategically throughout your code. Think of them as breadcrumbs, tracing the execution flow and pinpointing where things go awry. Imagine a maze: logs illuminate the path, highlighting dead ends and leading you to the treasure chest of understanding.
3. DevTools Dojo Master
Don’t underestimate the power of browser developer tools. Your browser harbors a hidden arsenal of debugging weapons. The debugger is your time-travel machine, allowing you to step through your code line by line, pausing at key moments to inspect variables and witness the code’s inner workings. Think of it as slowing down a bullet in mid-air, examining its composition before letting it fly again.
4. Divide and Conquer
Feeling overwhelmed by a mountain of spaghetti code? Don’t despair! Channel your inner Alexander the Great and divide the problem into smaller, more manageable bites. Isolate the section suspected of harboring the bug and treat it like a separate battlefield. Focus your debugging power on this smaller terrain, then triumphantly reunite the conquered territories into a seamlessly functioning whole.
5. The Sherlock Holmes of Debugging
Don’t just copy-paste solutions from Google. Be a critical thinker! Analyze the logic behind the proposed fix and understand how it addresses the underlying issue. This builds your debugging intuition, equipping you to tackle future challenges without relying solely on external help. Think of it as learning the art of deduction, not just relying on second-hand clues.
6. Patience is a Zen Virtue
Debugging is a marathon, not a sprint. Don’t expect instant resolutions. Embrace the journey, and approach each challenge with persistence and a positive attitude. Every successful debug, no matter how small, strengthens your skills and builds confidence. Remember, even the Buddha didn’t achieve enlightenment overnight.
7. The Power of the Rubber Duck
Sometimes, the simplest solutions lie in fresh perspectives. Are you stuck on a particularly nasty bug? Grab a rubber duck (or any non-judgmental listener) and explain your code step-by-step. Verbalizing your thought process often triggers unexpected insights, revealing blind spots hidden in your solo mind. This collaborative debugging technique, popularized by Larry Wall, creator of Perl, taps into the power of externalization to reveal hidden truths.
8. Community is Key
Don’t suffer in silence! The web development community is brimming with helpful individuals. Seek advice from forums, chatrooms, and online communities. Share your debugging struggles, and learn from the experiences of others. Remember, collaboration breeds innovation, and a shared struggle often leads to triumphs.
9. Practice Makes Perfect
Debugging is a skill that grows with practice. Don’t wait for bugs to appear in production code. Challenge yourself with coding exercises and mini-projects. Deliberately inject bugs into your code and then practice tracking them down and eliminating them. This proactive approach hones your debugging muscles, turning you into a lean, mean, error-slaying machine.
10. The Zen Code
Finally, remember the true essence of Zen: finding calm amidst the chaos. Embrace the challenges of debugging, celebrate the incremental victories, and learn from every experience. As your debugging skills evolve, so too will your inner zen, transforming JavaScript jitters into moments of tranquil code refinement and elegant problem-solving.
Go forth, code warriors! Armed with these zen-powered debugging techniques, you can conquer even the most cryptic errors and write JavaScript that sings with clarity and grace. May your code be ever-flowing, your coffee mugs forever stain-free, and your journey through the JavaScript wonderland one of calm, confident creation.
Example:
Imagine you’re building a shopping cart application. When users add items, a price total should be updated. But, alas, the total remains stubbornly constant!
1. Embrace the Error: “Price total not updating? Interesting…” Analyze the error message: “TypeError: Cannot convert undefined to number.”
2. Your Console Concierge: Log messages to track the price calculation process.
console.log("Item price:", itemPrice);
console.log("Quantity:", quantity);
console.log("Total before calculation:", total);
3. DevTools Dojo Master: Step through the code, examining variable values.
4. Divide and Conquer: Isolate the total calculation function.
5. The Sherlock Holmes of Debugging: Research similar errors and understand their causes.
Solution:
The error indicates a variable is undefined. Tracing back, you discover itemPrice
is being retrieved from a product object, but that object hasn’t fully loaded yet.
Fix:
- Restructure code to ensure product data is available before price calculation.
- Implement a conditional check:
if (product) {
// Calculate total using product.price
} else {
console.log("Product data not loaded yet.");
}
Example 2:
Your interactive map fails to display markers.
Steps:
- Error Whisperer: Check the console for errors related to map API or marker placement.
- Console Concierge: Log messages to track map initialization and marker creation.
- DevTools Dojo Master: Inspect map object properties and events.
- Divide and Conquer: Isolate map initialization and marker placement code.
- Sherlock Holmes of Debugging: Research common map API errors and solutions.
Solution:
Troubleshooting reveals incorrect API key usage.
Fix:
- Replace the API key with the correct one.
Example 3:
Your form submission generates a “ReferenceError: formData is not defined.”
Steps:
- Error Whisperer: Analyze the error message, indicating a missing variable.
- Console Concierge: Log messages to check variable declaration and usage.
- DevTools Dojo Master: Inspect the scope of variables.
Solution:
Discover formData
is declared inside a conditional block, but used outside its scope.
Fix:
- Declare
formData
in a wider scope accessible to all relevant code sections.
Remember, debugging is an art, not a science. Embrace the journey, trust your instincts, and don’t be afraid to experiment. With practice and patience, you’ll master the art of JavaScript debugging and create code that flows with clarity and harmony.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!